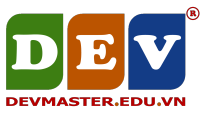
Mục tiêu
Phần I Bài tập step by step
Nhấn OK -> màn hình tiếp theo xuất hiện bạn chọn template là MVC sau đó chọn Change Authentication -> No Authentication -> OK -> OK.
public class Member
{
//với cách 1 và 2 ta sử dụng khai báo thuộc tính như sau
//public int? Id { get; set; }
//public string Username { get; set; }
//public string FullName { get; set; }
//public string Password { get; set; }
//public int? Age { get; set; }
//public string Email { get; set; }
//với cách 3 khai báo như sau:
[Required(ErrorMessage = "Hãy nhập mã số")]
[DataType(DataType.Currency)]
public int? Id { get; set; }
[Required(ErrorMessage = "Hãy nhập tên đăng nhập")]
public string Username { get; set; }
[Required(ErrorMessage = "Hãy nhập họ và tên")]
public string FullName { get; set; }
[Required(ErrorMessage = "Hãy nhập mật khẩu")]
[DataType(DataType.Password)]
public string Password { get; set; }
[Required(ErrorMessage = "Hãy nhập tuổi")]
[Range(18, 50, ErrorMessage = "Tuổi từ 18-50")]
public int? Age { get; set; }
[Required(ErrorMessage = "Hãy nhập email")]
[RegularExpression(@"[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}", ErrorMessage = "Email phải đúng định dạng")]
public string Email { get; set; }
}
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li>@Html.ActionLink("Sử dụng HTML5 validate", "CreateOne", "Member")</li>
<li>@Html.ActionLink("Tự validate", "CreateTwo", "Member")</li>
<li>@Html.ActionLink("Sử dụng Annotation validate", "CreateThree", "Member")</li>
</ul>
</div>
public class MemberController : Controller
{
// GET: /Member/CreateOne
public ActionResult CreateOne()
{
return View();
}
//POST: /Member/CreateOne
[HttpPost]
public ActionResult CreateOne(Member m)
{
//chuyển dữ liệu nhận được tới View Details
return View("Details",m);
}
//GET: /Member/CreateTwo
public ActionResult CreateTwo()
{
return View();
}
//POST: /Member/CreateTwo
[HttpPost]
public ActionResult CreateTwo(Member m)
{
//kiểm tra trống các trường và thông báo lỗi tới VIEW
if (m.Id==null)
{
ViewBag.error = "Hãy nhập mã số";
return View();
}
if (m.Username == null)
{
ViewBag.error = "Hãy nhập tên đăng nhập";
return View();
}
if (m.FullName == null)
{
ViewBag.error = "Hãy nhập tên họ và tên";
return View();
}
if (m.Age==null)
{
ViewBag.error = "Hãy nhập tuổi";
return View();
}
if (m.Email == null)
{
ViewBag.error = "Hãy nhập Email";
return View();
}
//mẫu kiểm tra Email
string regexPattern=@"[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}";
if (!System.Text.RegularExpressions.Regex.IsMatch(m.Email,regexPattern))
{
ViewBag.error = "Hãy nhập đúng định dạng";
return View();
}
//nếu không xảy ra lỗi thì chuyển dữ liệu tới View Details
return View("Details", m);
}
//GET: /Member/CreateThree
public ActionResult CreateThree()
{
return View();
}
//POST: /Member/CreateThree
[HttpPost]
public ActionResult CreateThree(Member m)
{
//nếu trạng thái dữ liệu của Model hợp lệ thì chuyển dữ liệu tới View Details
if (ModelState.IsValid)
return View("Details", m);
else
return View(); //quay lại view Three để báo lỗi
}
//GET: /Member/Details
public ActionResult Details()
{
return View();
}
}
Chỉnh lại code Razor theo gợi ý sau:
@model Lab05.Models.Member
@{
ViewBag.Title = "Chi tiết thành viên";
}
<div>
<h2>Chi tiết thành viên</h2>
<hr />
<dl class="dl-horizontal">
<dt>
Mã số
</dt>
<dd>
@Html.DisplayFor(model => model.Id)
</dd>
<dt>
Tên đăng nhập
</dt>
<dd>
@Html.DisplayFor(model => model.Username)
</dd>
<dt>
Họ và tên
</dt>
<dd>
@Html.DisplayFor(model => model.FullName)
</dd>
<dt>
Mật khẩu
</dt>
<dd>
@Html.DisplayFor(model => model.Password)
</dd>
<dt>
Tuổi
</dt>
<dd>
@Html.DisplayFor(model => model.Age)
</dd>
<dt>
</dt>
<dd>
@Html.DisplayFor(model => model.Email)
</dd>
</dl>
</div>
<p>
@Html.ActionLink("Quay lại", "CreateOne")
</p>
Chỉnh lại code Razor theo gợi ý sau:
@model Lab05.Models.Member
@{
ViewBag.Title = "Sử dụng HTML validate data";
}
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h2>Thêm thành viên</h2>
<hr />
<div class="form-group">
@Html.Label("Mã thành viên", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.TextBoxFor(model => model.Id, new { Required = "Required", type="number" })
</div>
</div>
<div class="form-group">
@Html.Label("Tên đăng nhập", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.TextBoxFor(model => model.Username, new {Required="Required" })
</div>
</div>
<div class="form-group">
@Html.Label("Họ và tên", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.TextBoxFor(model => model.FullName, new {Required="Required" })
</div>
</div>
<div class="form-group">
@Html.Label("Mật khẩu", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.PasswordFor(model => model.Password, new { Required = "Required" })
</div>
</div>
<div class="form-group">
@Html.Label("Tuổi", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.TextBoxFor(model => model.Age, new { Required = "Required", type = "number", min="18", max="60" })
</div>
</div>
<div class="form-group">
@Html.Label("Email", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.TextBoxFor(model => model.Email, new { Required = "Required", type = "email" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value=" Lưu " class="btn btn-default" />
</div>
</div>
</div>
}
@model Lab05.Models.Member
@{
ViewBag.Title = "Kiểm tra dữ liệu theo cách 2";
}
<h2>Kiểm tra dữ liệu theo cách 2</h2>
@if(ViewBag.error!=null)
{
<div class="alert alert-danger">@ViewBag.error</div>
}
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
<div class="form-group">
@Html.Label("Mã thành viên", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Id)
</div>
</div>
<div class="form-group">
@Html.Label("Tên đăng nhập", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Username)
</div>
</div>
<div class="form-group">
@Html.Label("Họ và tên", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.FullName)
</div>
</div>
<div class="form-group">
@Html.Label("Mật khẩu", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.PasswordFor(model => model.Password)
</div>
</div>
<div class="form-group">
@Html.Label("Tuổi", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Age)
</div>
</div>
<div class="form-group">
@Html.Label("Email", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Email)
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value=" Lưu " class="btn btn-default" />
</div>
</div>
</div>
}
@model Lab05.Models.Member
@{
ViewBag.Title = "Kiểm tra dữ liệu theo cách 3";
}
<h2>Kiểm tra dữ liệu theo cách 3</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
@Html.ValidationSummary(true)
<div class="form-group">
@Html.Label("Mã thành viên", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Id)
@Html.ValidationMessageFor(model => model.Id)
</div>
</div>
<div class="form-group">
@Html.Label("Tên đăng nhập", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Username)
@Html.ValidationMessageFor(model => model.Username)
</div>
</div>
<div class="form-group">
@Html.Label("Họ và tên", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.FullName)
@Html.ValidationMessageFor(model => model.FullName)
</div>
</div>
<div class="form-group">
@Html.Label("Mật khẩu", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Password)
@Html.ValidationMessageFor(model => model.Password)
</div>
</div>
<div class="form-group">
@Html.Label("Tuổi", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Age)
@Html.ValidationMessageFor(model => model.Age)
</div>
</div>
<div class="form-group">
@Html.Label("Email", new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Email)
@Html.ValidationMessageFor(model => model.Email)
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value=" Lưu " class="btn btn-default" />
</div>
</div>
</div>
}
Nguồn: Devmaster Academy